ShowTable of Contents
XPages2Eclipse control in DDE
After you have successfully
installed the XPages2Eclipse product in your Lotus Notes/Domino environment, a new control shows up in the control palette of the XPages editor in Domino Designer (DDE):
To use XPages2Eclipse functionality in an XPage of your application, simply drag this control to the XPage. The first time you do this, you will see this prompt that you need to confirm:
API access from server-side JavaScript (SSJS)
To open a connection to the API and call a test method, add this code snippet to server-side JavaScript (SSJS) code, e.g. in the onClick event of a button:
var conn=X2E.createConnection();
var platformUI=com.x2e.PlatformUIAPI.getUI(conn);
platformUI.logToStatusBar("Hello World!");
The complete XML markup for this page would look like this:
<?xml version="1.0" encoding="UTF-8"?>
<xp:view xmlns:xp="http://www.ibm.com/xsp/core"
xmlns:xpages2eclipse="http://www.mindoo.com/x2e">
<xpages2eclipse:apiinit id="apiinit1"></xpages2eclipse:apiinit>
<xp:br></xp:br>
<xp:button value="Test" id="button1">
<xp:eventHandler event="onclick" submit="true"
refreshMode="complete">
<xp:this.action><![CDATA[#{javascript:var conn=X2E.createConnection();
var platformUI=com.x2e.PlatformUIAPI.getUI(conn);
platformUI.logToStatusBar("Hello World!"); }]]></xp:this.action>
</xp:eventHandler>
</xp:button>
</xp:view>
The method
createConnection
creates a new API connection that is only valid for the current XPages page request. The connection is automatically disposed and it is the entry point for any API method call. Multiple calls of
X2E.createConnection()
return the same connection instance for the current XPages request, the calls do not add performance overhead.
Line 2 of our snippet accesses the first API that we will get to know: the PlatformUI API. This API corresponds to the Eclipse API class with
the same name
, with some functionality modified, added and removed to make it usable from an XPages application environment. PlatformUI gives you access to the Notes client's "workbench", it lets you switch and create Eclipse "perspectives" (shown as main tabs in Lotus Notes) and add/remove Eclipse "viewparts" (the areas with border and title bar you probably know from Composite Applications).
logToStatusBar
is not actually part of the Eclipse version of PlatformUI, but was added to quickly write some status and debug information to the Notes Client status bar, which would be much more difficult when using Eclipse API directly.
API access from Java
If you prefer to write the backend code of your XPage in Java instead of SSJS, the syntax is quite similar:
package com.mindoo.x2esamples.helloworld;
import com.mindoo.remote.api.IRemoteEclipseConnection;
import com.mindoo.remote.api.RemoteEclipseAPIException;
import com.mindoo.remote.api.org.eclipse.ui.IRemotePlatformUI;
import com.mindoo.xpages2eclipse.X2E;
public class HelloWorld {
public void doCallAPI(String username) throws RemoteEclipseAPIException {
IRemoteEclipseConnection conn=X2E.createConnection();
IRemotePlatformUI platformUI=com.x2e.PlatformUIAPI.getUI(conn);
platformUI.logToStatusBar("Java: Hello "+username+"! How are you?");
}
}
Detect if API is available and check version
The Notes Client and Domino Server HTTP process will display an error message, when the XPages2Eclipse plugin is not installed at all. This is caused by a technical plugin dependency check of Notes/Domino.
But even if the plugin is installed, you often need to check if the API implementation is available. For example, on the web, calling any of the Notes Client API methods would produce errors, because there are no Eclipse APIs that we could use.
Here is a snippet that you can use to check the API version and it's availability:
boolean flag=X2E.isAvailable(); //returns true if API methods can be used
String version=X2E.getAPIVersion(); //returns a string like 1.0.0.201104052048
You can use both methods to hide specific functionality in your XPages application if your code is running on the web:
<xp:label value="API is *not* available. Run this app in the Notes Client!" id="label1"
style="color:rgb(0,128,0)" rendered="#{javascript:!X2E.isAvailable();}"></xp:label>
Sample application
The sample application attached to this article contains a simple XPage that writes the name of the user to the Client's status bar:
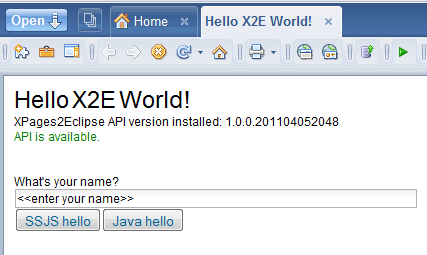
Full API class reference
Please refer to the following articles for a complete Javadoc reference of the API classes for XPages2Eclipse and Add-on packages:
XPages2Eclipse Base API Javadocs